Odds and Ends
Android Studio View Container, 안드로이드 뷰 컨테이너 구현 방법(실습) 본문
01 간단한 기능의 뷰 컨테이너
* 스크롤 뷰
- XML 코드 자체로 구성이 가능함
- 위젯이나 레이아웃이 화면에 넘칠 떄 스크롤 효과를 낼 수 있음
- 스크롤뷰는 수직(위아래)으로 스크롤 하는 기능
: 수평(좌우)으로 스크롤하는 수평 스크롤뷰(HorizontalScrollView)는 따로 존재
- 스크롤 뷰에는 단 하나의 위젯만 넣을 수 있음
: 주로 스크롤뷰 안에 리니어레이아웃을 1개 넣고, 리니어 레이아웃 안에 자신이 원하는 것을 여러개 넣는 방법을 사용함
* 슬라이딩드로어(SlidingDrawer)
- 위젯을 서랍처럼 열어서 보여주거나 닫아서 감춤
- 슬라이딩드로어의 규칙
: 슬라이딩 드로어의 handle 속성에 지정된 이름과 슬라이딩 드로어의 손잡이 역할을 하는 버튼의 id가 동일해야함
: 버튼 대신 이미지뷰나 이미지 버튼으로 사용해도 상관 없음
: 슬라이딩 드로어의 content 속성에 지정된 이름과 리니어 레이아웃의 id도 동일해야함
: 리니어 레이아웃이 아닌 다른 레이아웃도 가능함
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="여기는 서랍 밖입니다."
android:textSize="20dp"/>
<SlidingDrawer
android:id="@+id/slidingDrawer1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:content="@+id/content"
android:handle="@+id/handle" >
<Button
android:id="@+id/handle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="서랍 손잡이" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/content"
android:background="#00ff00"
android:gravity="center" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="여기는 서랍 안입니다."
android:textSize="20dp"/>
</LinearLayout>
</SlidingDrawer>
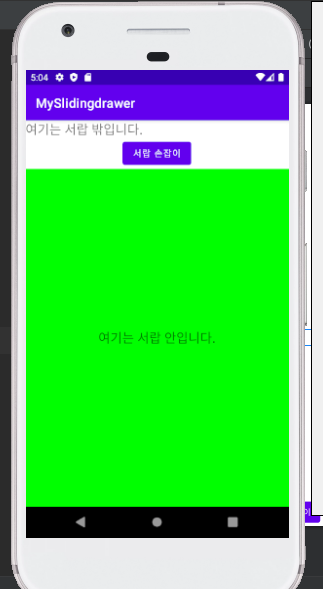
예제 실행 시
* 뷰 플리퍼
- 안에 여러 개의 위젯을 배치하고 필요에 따라 화면을 왼쪽 또는 오른쪽으로 밀어서 위젯을 하나씩 보여주는 방식의 뷰 컨테이너
- 한 번에 보유줄 위젯이 여러개라면 뷰플리퍼 안에 레이아웃을 여러 개 넣고 각 레이아웃에 필요한 위젯을 배치함
btnPrev.setOnClickListener{
vFlipper.showPrevious()
}
btnNext.setOnClickListener {
vFlipper.showNext()
}
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btnPrev"
android:layout_weight="1"
android:text="이전화면"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btnNext"
android:layout_weight="1"
android:text="다음화면"/>
</LinearLayout>
<ViewFlipper
android:id="@+id/viewFlipper1"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ff0000" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffff00" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#00ff00" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#00ffff" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0000ff" >
</LinearLayout>
</ViewFlipper>
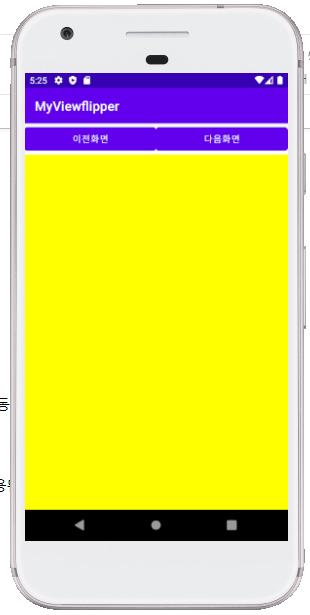
예제 실행 화면
버튼 클릭시 화면 색상 바뀐다(리니어 레이아웃 당 배경화면 다르게 했음)
02 복잡한 기능의 뷰 컨테이너
* 프래그먼트
- 안드로이드의 액티비티는 화면을 표현하기 위한 기본 단위
- 프래그먼트는 화면을 분할해서 독립적인 코드로 구성할 수 있게 도와주는 것
: 허니콤에서 소개된 기능
: 액티비티보다 작은 단위의 화면
: Fragment는 Activity와 View 사이에 존재하는 레이어의 개념으로 생각하면 이해하기 쉽다.
: Activity-Fragment-View 구조를 사용하면 오른쪽 그림의 예에서 초록색 Fragment를 제거하고 동적으로 다른 Fragment를 새롭게 추가하는 등의 작업을 자유롭게 수행 가능
: 대형 화면에서 액티비티 화면을 분할하여 표현 가능
: 소형 화면에서는 화면의 분할보다는 실행중에 화면을 동적으로 추가하거나 제거하는데 더 많이 활용됨
* 뷰 페이저
- 이전, 현재, 다음 세개의 뷰를 준비해 놓고 하나씩 넘겨보는 구조
: 첫번째일 때는 1번, 2번, 두번째일 떄는 1번 2번3번, 세번째일 때는 2,3,4번의 뷰를 가지고 있음
: 그 외의 pager는 자동적으로 삭제
- 뷰를 많이 붙이면 성능 저하, 특히 이미지가 포함된 뷰가 많은 경우엔 ViewFlipper보다 ViewPager가 효율적임
- 뷰가 바뀔 떄 만화책의 Page를 넘기는 듯 손가락으로 드래그해서 자염스럽게 넘어가는 Animation을 볼 수 있음(Swipe)